Jakarta POIは、
Javaを使ってマイクロソフトのWord文書や、Excelワークシートを作成したり、
読み書きしたりするAPIのライブラリです。
この「Javaを使ってExcelシートが生成できる」というのは、
例えば
「Webアプリケーションでデータベースからデータを抽出し、
Excel形式で保存して、エンドユーザに配布する」
といった応用が考えられ、とても興味深いものがあります。
若干複雑ですが日本語の文字列をセルやシート名に使うこともできますし、
フォントに「MS ゴシック」など日本のTrueTypeフォント名を指定することも可能です。
と・いうわけで、このページではPOIのquick-startガイドを真似て作った、
簡単なサンプルをご紹介したいと思います。
●インストール
上記のホームページから、ダウンロードサイトの「dev」ディレクトリへ行き、
「jakarta-poi-1.10.0-dev-bin.tar.gz」をダウンロードし、展開します。
中に入っているjakarta-poi-1.10.0-dev-bin.jar
をCLASSPATHに加えます。
●空のブックを作る
まず、中身が空のブックを作って、カレントディレクトリに出力するプログラムです。
2番目の例(PoiExample2.java)では、2つのシートを持ったブックが出力されます。
/**
* PoiExample1.java
* 空のワークブックを作成します。
*/
import java.io.*;
import org.apache.poi.hssf.usermodel.*;
public class PoiExample1 {
public static void main(String[] args) throws Exception {
HSSFWorkbook wb = new HSSFWorkbook();
FileOutputStream fileOut = new FileOutputStream("workbook.xls");
wb.write(fileOut);
fileOut.close();
}
}
/* end. */
|
import java.io.*;
import org.apache.poi.hssf.usermodel.*;
public class PoiExample2 {
public static void main(String[] args) throws Exception {
HSSFWorkbook wb = new HSSFWorkbook();
HSSFSheet sheet1 = wb.createSheet("new sheet");
HSSFSheet sheet2 = wb.createSheet("second sheet");
FileOutputStream fileOut = new FileOutputStream("workbook.xls");
wb.write(fileOut);
fileOut.close();
}
}
/* end. */
|
●セルを操作する
今度は、空のブックを作り、セルに値をセットしたり、セルの書式を変更したりするサンプルです。
import java.io.*;
import java.util.Date;
import org.apache.poi.hssf.usermodel.*;
public class PoiExample3 {
public static void main(String[] args) throws Exception {
HSSFWorkbook wb = new HSSFWorkbook();
//
// 日本語はそのまま記述できません。
HSSFSheet sheet = wb.createSheet("new_sheet");
// Create a row and put some cells in it. Rows are 0 based.
// キャストの(short)は省略できません。
HSSFRow row = sheet.createRow((short)0);
// Create a cell and put a date value in it. The first cell is not styled
// as a date.
HSSFCell cell = row.createCell((short)0);
cell.setCellValue(new Date());
// we style the second cell as a date (and time). It is important to
// create a new cell style from the workbook otherwise you can end up
// modifying the built in style and effecting not only this cell but other cells.
HSSFCellStyle cellStyle = wb.createCellStyle();
cellStyle.setDataFormat(HSSFDataFormat.getBuiltinFormat("m/d/yy h:mm"));
//cellStyle.setDataFormat(HSSFDataFormat.getFormat("m/d/yy h:mm"));
cell = row.createCell((short)1);
cell.setCellValue(new Date());
cell.setCellStyle(cellStyle);
// 同じ値(12345.67)を、書式を変えて貼り付けてみます。
{
// 整数(小数点以下四捨五入)になります。
double sampleValue = 12345.67;
HSSFCellStyle cs = wb.createCellStyle();
cs.setDataFormat(HSSFDataFormat.getBuiltinFormat("0"));
HSSFCell c = row.createCell((short)5);
c.setCellValue(sampleValue);
c.setCellStyle(cs);
}
{
double sampleValue = 12345.67;
HSSFCellStyle cs = wb.createCellStyle();
cs.setDataFormat(HSSFDataFormat.getBuiltinFormat("0.00"));
HSSFCell c = row.createCell((short)6);
c.setCellValue(sampleValue);
c.setCellStyle(cs);
}
{
// 書式が@(文字列)だと、数値としてセットしても文字列としてセットしても、
// 文字列(左詰)になります。
double sampleValue = 12345.67;
HSSFCellStyle cs = wb.createCellStyle();
cs.setDataFormat(HSSFDataFormat.getBuiltinFormat("@"));
HSSFCell c = row.createCell((short)7);
c.setCellValue(sampleValue);
c.setCellStyle(cs);
}
{
double sampleValue = 12345.67;
HSSFCellStyle cs = wb.createCellStyle();
cs.setDataFormat(HSSFDataFormat.getBuiltinFormat("General"));
HSSFCell c = row.createCell((short)9);
c.setCellValue(sampleValue);
c.setCellStyle(cs);
}
// Write the output to a file
FileOutputStream fileOut = new FileOutputStream("workbook.xls");
wb.write(fileOut);
fileOut.close();
}
}
/* end. */
|
/**
* PoiExample4.java
* 枠線を引きます。
*/
import java.io.*;
import org.apache.poi.hssf.util.*;
import org.apache.poi.hssf.usermodel.*;
public class PoiExample4 {
public static void main(String[] args) throws Exception {
HSSFWorkbook wb = new HSSFWorkbook();
HSSFSheet sheet = wb.createSheet("new sheet");
// Create a row and put some cells in it. Rows are 0 based.
HSSFRow row = sheet.createRow((short) 1);
// Create a cell and put a value in it.
HSSFCell cell = row.createCell((short) 1);
// セルの値をセット
//cell.setCellValue(43.21);
//cell.setCellValue("Hello World");
cell.setCellValue("00123");
// 「00123」も先頭の00が削られず、そのままセットされます。
// Style the cell with borders all around.
HSSFCellStyle style = wb.createCellStyle();
// セルの下部(黒で、細い線)
style.setBorderBottom(HSSFCellStyle.BORDER_THIN);
style.setBottomBorderColor(HSSFColor.BLACK.index);
// セルの左側(緑で、細い線)
style.setBorderLeft(HSSFCellStyle.BORDER_THIN);
style.setLeftBorderColor(HSSFColor.GREEN.index);
// セルの青側(緑で、細い線)
style.setBorderRight(HSSFCellStyle.BORDER_THIN);
style.setRightBorderColor(HSSFColor.BLUE.index);
// 塗りつぶし色の設定
style.setFillForegroundColor(HSSFColor.SEA_GREEN.index);
style.setFillPattern(HSSFCellStyle.SOLID_FOREGROUND);
// セルの上部(黒、普通の太さの点線)
style.setBorderTop(HSSFCellStyle.BORDER_MEDIUM_DASHED);
style.setTopBorderColor(HSSFColor.BLACK.index);
cell.setCellStyle(style);
// Write the output to a file
FileOutputStream fileOut = new FileOutputStream("workbook.xls");
wb.write(fileOut);
fileOut.close();
}
}
/* end. */
|
できたばかりのブックは、0行0列のセル・オブジェクトを持っている状態になっています。
セルに値をセットするには、
まず行がなければ作り、さらにセルがなければその行の上に作ります。
これは、
HSSFRow row = sheet.createRow((short)0);
HSSFCell cell = row.createCell((short)0);
cell.setCellValue(12345);
|
こんな感じで行えます。列、行はshortで指定し、このキャスト(型変換)は省略できません。
列、行とも、左上を0として数え始めます。
セルに書式をセットしたり、枠線をつけたりすることも可能です。
書式を文字列にするには、サンプルに示すように書式(DataFormat)を「@」
にする方法がありますが、それと別に、下のように「タイプ」で指定することも出来ます。
HSSFCell c = row.createCell((short)8);
c.setCellType(HSSFCell.CELL_TYPE_STRING);
|
cell.setCellValueの引数でセルの値をセットするときに、数値(intやdouble)で指定するか、
文字列で指定するかは、Excelで見たときに違いはないようです。
Excel的に数値を判断されるか、文字列と判断されるかは、
あくまで書式によるみたいです。
またおまけですが、書式を文字列にしておけば、setCellValueメソッドで「00123」
のように0左詰の文字列を指定しても、0が削られずにそのまま「00123」
という形でセットしてくれます。
●既存のExcelシートを読む
今度は既存のブックを開いて、その一部を編集するサンプルです。
簡単なのでコードを見て頂ければ大体把握できると思います。
/**
* PoiExample5.java
* 既存のファイルを開き、一部を変更して書き出します。
*/
import java.io.*;
import org.apache.poi.hssf.util.*;
import org.apache.poi.poifs.filesystem.*;
import org.apache.poi.hssf.usermodel.*;
public class PoiExample5 {
public static void main(String[] args) throws Exception {
POIFSFileSystem fs =
new POIFSFileSystem(new FileInputStream("workbook.xls"));
HSSFWorkbook wb = new HSSFWorkbook(fs);
HSSFSheet sheet = wb.getSheetAt(0);
HSSFRow row = sheet.getRow(2);
if(row == null){
System.out.println("行がまだ作られていないので、作ります。");
row = sheet.createRow( (short) 2);
}
else{
System.out.println("行は既に作られています。");
}
HSSFCell cell = row.getCell((short)3);
if(cell == null){
cell = row.createCell((short)3);
}
cell.setCellType(HSSFCell.CELL_TYPE_STRING);
cell.setCellValue("a test");
// Write the output to a file
FileOutputStream fileOut = new FileOutputStream("workbook.xls");
wb.write(fileOut);
fileOut.close();
}
}
/* end. */
|
●日本語を使う
そして最後に、シートの名前、セルの値、またフォントに日本語を使った例です。
これはPOIの古いバージョンでは使えないことがあるので注意が必要です。
import java.io.*;
import java.util.Date;
import org.apache.poi.hssf.usermodel.*;
/**
* 日本語の入力を試します。
*/
public class PoiExample6 {
public static void main(String[] args) throws Exception {
HSSFWorkbook wb = new HSSFWorkbook();
// 日本語入力を試す
HSSFSheet sheet = wb.createSheet("dummy");
wb.setSheetName(0, "新しいシート", HSSFWorkbook.ENCODING_UTF_16);
//wb.setSheetName(0, "new_sheet");
// Create a row and put some cells in it. Rows are 0 based.
// キャストの(short)は省略できません。
HSSFRow row = sheet.createRow((short)0);
// Create a cell and put a date value in it. The first cell is not styled
// as a date.
HSSFCell cell = row.createCell((short)0);
cell.setCellValue(new Date());
// we style the second cell as a date (and time). It is important to
// create a new cell style from the workbook otherwise you can end up
// modifying the built in style and effecting not only this cell but other cells.
HSSFCellStyle cellStyle = wb.createCellStyle();
cellStyle.setDataFormat(HSSFDataFormat.getBuiltinFormat("m/d/yy h:mm"));
//cellStyle.setDataFormat(HSSFDataFormat.getFormat("m/d/yy h:mm"));
cell = row.createCell((short)1);
cell.setCellValue(new Date());
cell.setCellStyle(cellStyle);
{
// 日本語入力を試す
HSSFCell c = row.createCell((short)8);
c.setCellType(HSSFCell.CELL_TYPE_STRING);
c.setEncoding(HSSFCell.ENCODING_UTF_16);
HSSFFont font = wb.createFont();
font.setFontHeightInPoints((short)24);
//font.setFontName("Courier New");
font.setFontName("MS ゴシック");
// ↑日本語のフォント名もそのまま指定できます(Sとゴの間は半角空白です)
HSSFCellStyle style = wb.createCellStyle();
style.setFont(font);
c.setCellValue("ハロー, POIワールド。で日本語を試す。");
c.setCellStyle(style);
}
// Write the output to a file
FileOutputStream fileOut = new FileOutputStream("workbook.xls");
wb.write(fileOut);
fileOut.close();
}
}
/* end. */
|
ワークシートの名前に日本語を使うには、
HSSFWorkbook.setSheetNameメソッドの3番目の引数に、
記号定数 HSSFWorkbook.ENCODING_UTF_16 を指定します。
また、セルの値に日本語をセットするには、
HSSFCell c = row.createCell((short)8);
c.setCellType(HSSFCell.CELL_TYPE_STRING);
c.setEncoding(HSSFCell.ENCODING_UTF_16);
c.setCellValue("日本語");
|
このように、setEncodingメソッドを使います。
最後に、日本語のTrueTypeフォント名ですが、
これはサンプルのように、直接フォント名で指定できます。
font.setFontName("MS ゴシック");
// ↑日本語のフォント名もそのまま指定できます(Sとゴの間は半角空白です)
|
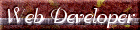
(first uploaded 2003/07/12 last updated 2003/11/03, URANO398)
|